Home / Blog / Why should you use TypeScript?
Why should you use TypeScript?
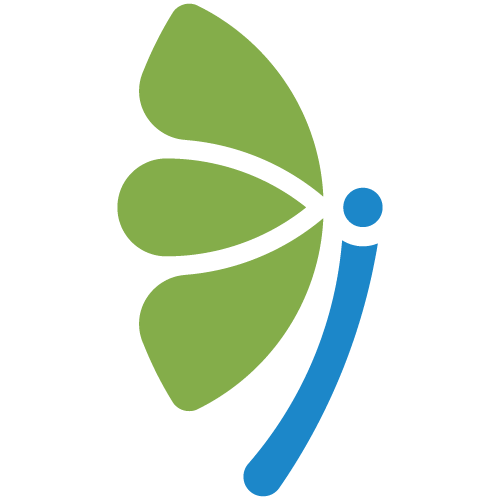
By EKbana on December 21, 2022
10m readPeople say JavaScript is the most hated programming language in the world but do you know what the most loved and popular language in the world is? Its JavaScript. I feel like many people hate and become frustrated when they get their first hands-on to JavaScript because they don't understand JavaScript. It is very important to understand the core fundamentals of JavaScript before saying that things are not working the way you are expecting while executing different programs in JavaScript. However, bugs in programming are just unavoidable.
As we know that JavsScript is a dynamically typed language, it does not have a data type like regular C or Java and a single variable can either contain text, a number, or even a whole database entity, depending on the situation - and that is just fine. The strength and weaknesses of JavaScript are that it is dynamic. It is very easy to change properties because there are so many objects that cannot be tracked.
This often leads to bugs that not only decrease the efficiency of the developer’s work but also bring development to halt due to the increasing costs of adding new lines of code. JavaScript could be a bad choice for server-side code in enterprises and large codebases because it failing to incorporate things like types and compile-time error checks. This is where TypeScript comes into the picture.
Why TypeScript then?
TypeScript is basically JavaScript with additional features such as compilation, static typing, and Object Oriented Programming. It is a superset of typed JavaScript that can help build and manage large-scale JavaScript projects.
TypeScript is particularly useful for larger projects where identifying a small “developer error” (like a typo or an edge case) can become time-consuming and painful. It will be worth mentioning that in the end TypeScript compiles to JavaScript so the final code can be run on any environment whether that's client-side or server-side which supports JavaScript.
According to researchers, TypeScript detects 15% of common errors at the compile stage. Although this is far from a 100% result, this is still a significant amount to let developers focus on resolving logical errors rather than making them stuck catching common bugs in their code.
Let’s consider I have a program below which basically tries to multiply strings and objects together. I would get an error in JavaScript if I try to run this code because there is not really any way to multiply a string and an object together.

But, in TypeScript, we can prevent any type-related errors from creeping into our project without even having to run the code, and considering the above scenario, I would get an error when I try to compile this code to JavaScript saying “The left-hand side of an arithmetic operation must be of type ‘any’, ‘number’, ‘bigint’ or an enum type”.

TypeScript is also very good at automatically inferring the types of new variables. When we say const firstName = ‘prajwal’, it knows that firstName is a string because of the value I assigned to. In these cases, when writing a “script”, the TypeScript compiler can help prevent errors.
Here are some good reasons I feel why you should use TypeScript.
Object Oriented Programming (OOP)
TypeScript supports OOP concepts such as Classes, inheritances, interfaces, Method Access Modifiers, and so on. which makes it easier to build well-organized, robust, clean, and scalable code. This advantage becomes more obvious when our project grows in size and complexity.

Interfaces
They are a good way of specifying a contract.

Generics
They help to provide compile-time checking.

Inheritance
They enable new objects to take on the properties of existing objects..

Access Modifiers
They control the accessibility of the members of a class. It has 2 access modifiers - public and private. By default, the members are public but we can explicitly add a public or private modifier to them.

Optional Static Typing
Let's imagine we have a really complicated banking system that distributes interests every quarter to their customers automatically. So in vanilla JavaScript, this processing function may look like this:

If our system uses the above function to add up interests in the principal amount of their customers and update their accounts automatically. We are assuming here that this function is taking numbers as arguments and should work without any issues flawlessly. However, due to some issues in our backend, we are getting a list of interests as strings instead of numbers. Can you guess what could possibly go wrong here? Let's see with an example:

The result is 25000 as a string instead of 2500 as a number. The customers would be really happy that they got an additional 0 at the end of their principal amount instead of getting no interest at all. The above program can be implemented in TypeScript in the following way:

Hence we know that in JS (dynamically typed language) there is no built-in static typing, so even if we create this function intending to add numbers, JS would not know that, and unexpected scenarios can take place. But in typescript, we can explicitly define the function parameter type which prevents us from passing the wrong type of argument to the function and prevent certain issues already at the software development stage. The biggest system can be broken by the smallest bug and typescript helps to fix this issue.
Static typing is not only about catching bugs but also gives the code more structure, makes it more readable and self-documenting, and speeds up debugging and refactoring. It increases productivity across a large team. TypeScript does not force us to use types everywhere so we have that flexibility and we are free to change the level of type strictness in different parts of the application.
Readability - TypeScript documents itself
Typescript is more readable in comparison with JavaScript because of the addition of strict types and other elements such as interfaces, and classes that make the code more self-expressive, meaningful, and easy to read and understand. This way we can see the design intent of our team members when we are working in large teams on the same project.
Let's assume that we have a function called renderProducts which takes products as an argument.

If we want to know what “products” are in JavaScript, it can be time-consuming and confusing at the same time, but there are a few options:
- If exists, we can read the documentation about this function.
- We can console.log(products); and run the application to check the “products” arguments.
- We can ask our teammates if someone has been recently working on it.
- We can track what data is put onto it after finding where the function is used.
- Though this is not the best idea, we can just assume that the product's argument is what we think it is.
With all these options to find out what we are looking at, in a complicated codebase, it would be really difficult to stop what that argument is and what type of data it contains. But we typescript, we can see what that argument is and what type of data it holds directly in our IDE or the compiler when something goes wrong. The same function above would look like the following in typescript:

Without even reading the whole function definition, we can know that the argument of the product is a product type array and what the product consists of.
Compatibility
After the TypeScript compiler translates the TS code into vanilla JS, every device, platform, or browser that runs JS also works with TS. Usually, a built-in TS compiler is present in the IDEs and editors supporting TypeScript. Similarly, it’s fully compatible with ES6. This assures to have smooth switching and language portability.
Rich IDE Support
We, developers, spend most of our time in some IDEs such as IntelliJ IDEA or lighter code editors VSCode writing different programs. These IDEs make our lives easier and faster rather than writing code in a simple notepad which would be more painful and can consume far more time. Some features that Typescript provides include mouse hover support, code auto-completion, real-time type checking, easier code refactoring, and much more.
Final Thoughts
A typeScript is a great tool for you to get started even if you don't want to use it to its full capacity. You can always learn, start small, and grow slowly by utilizing new features that TypeScript has to offer. Here is the basic migration example from your existing JavaScript file to TypeScript. Technically every JS file is a valid TS file, so we can just switch the file extension from .js to .ts.

The above code is valid JavaScript or TypeScript but if we have to only sum numbers, we can add a type signature to multiply the function to make it accept only numbers. In this case, the typescript provides us with an error saying: “Argument of type ‘string’ is not assignable to parameter of type ‘number’.”.

We know this error even before compiling the program which is really handy to find out these small bugs which we could have easily missed or even ignored in the core javascript.
I hope this article gives you some insights into why you should use typescript in your future applications.
Happy Learning! Happy Coding!